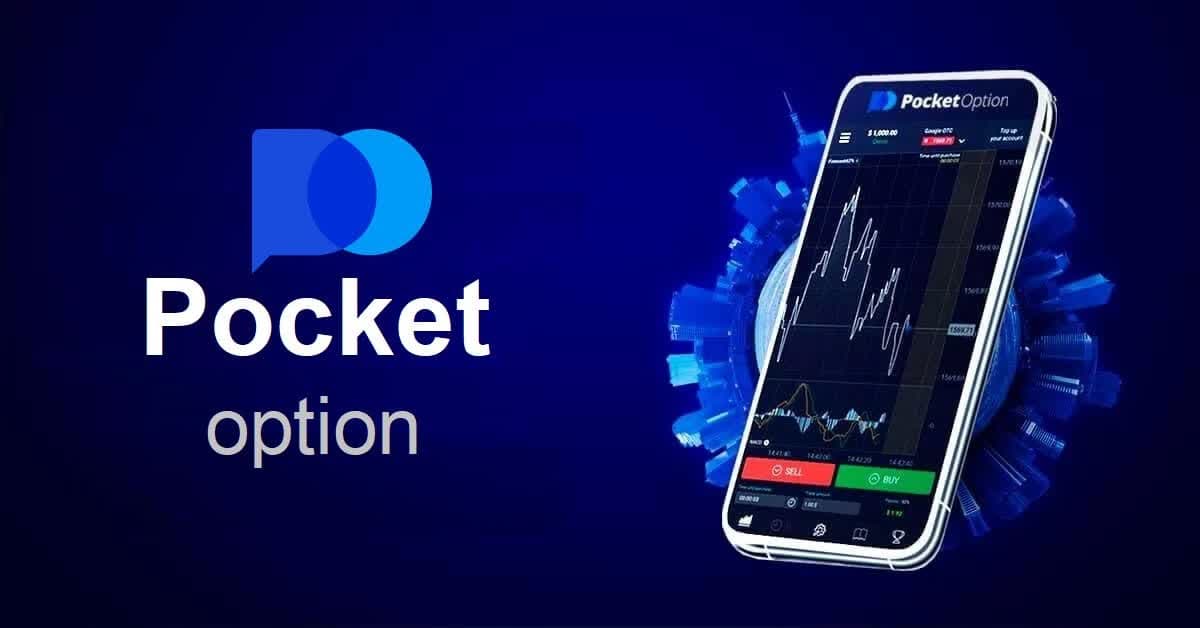
Mastering Your Trades with Pocket Option API Python
The pocket option api python pocket option api python is a powerful tool that transforms how traders manage their binary options strategies. With the rapid advancement of technology, the need for automation in trading has become increasingly apparent, and Python offers an excellent platform for achieving this goal. This article will explore how to get started with the Pocket Option API using Python, allowing traders to execute trades, retrieve market data, and implement their trading strategies with greater efficiency.
What is Pocket Option?
Pocket Option is a popular online trading platform that allows users to trade binary options on various assets, including forex, stocks, and cryptocurrencies. The platform offers a user-friendly interface that is appealing to both novice and experienced traders. In addition to its easy-to-use trading tools, Pocket Option provides an API that enables developers to automate trading processes. This API allows users to integrate Pocket Option’s capabilities into their own applications, making trading more accessible and efficient.
Understanding the Pocket Option API
The Pocket Option API offers various endpoints that allow users to perform a wide range of operations, such as retrieving market data, executing trades, managing accounts, and monitoring trading performance. The API is RESTful, which means it adheres to standard HTTP methods, making it easy to interact with using Python’s robust libraries for HTTP requests.
Authentication
Before you can start using the Pocket Option API, you need to authenticate your access. This is typically done using an API key provided by Pocket Option when you create an account. Make sure to keep your API key secure, as it grants access to your trading account.
Getting Started with Python
To begin using the Pocket Option API with Python, you will need to install some essential libraries. The two main libraries you will require are requests for making HTTP requests and json for handling JSON data.
pip install requests
Example: Connecting to the API
Once you have your API key and the necessary libraries installed, you can start writing Python code to connect to the API. Below is a simple example that demonstrates how to authenticate and make a basic request to retrieve account information:
import requests
import json
API_KEY = 'your_api_key_here'
BASE_URL = 'https://api.pocketoption.com/v1'
headers = {
'Authorization': f'Bearer {API_KEY}',
}
# Function to get account information
def get_account_info():
response = requests.get(f'{BASE_URL}/account', headers=headers)
if response.status_code == 200:
return response.json()
else:
raise Exception(f'Error: {response.status_code}, Message: {response.text}')
# Fetch account information
account_info = get_account_info()
print(json.dumps(account_info, indent=4))
Executing Trades
After you have successfully connected to the API and retrieved your account information, you can start executing trades. The Pocket Option API allows you to place trades by sending POST requests to the appropriate endpoint. Here’s an example of how to execute a trade:
def place_trade(asset, direction, amount):
payload = {
'asset': asset,
'direction': direction,
'amount': amount,
}
response = requests.post(f'{BASE_URL}/trade', headers=headers, json=payload)
if response.status_code == 200:
return response.json()
else:
raise Exception(f'Error: {response.status_code}, Message: {response.text}')
# Example trade
trade_response = place_trade('BTCUSD', 'call', 10)
print(json.dumps(trade_response, indent=4))
Retrieving Market Data
In addition to executing trades, the Pocket Option API allows you to retrieve market data for various assets. This data is critical for making informed trading decisions. Below is an example that demonstrates how to fetch market quotes:
def get_market_data(asset):
response = requests.get(f'{BASE_URL}/market/{asset}', headers=headers)
if response.status_code == 200:
return response.json()
else:
raise Exception(f'Error: {response.status_code}, Message: {response.text}')
# Fetch market data for BTCUSD
market_data = get_market_data('BTCUSD')
print(json.dumps(market_data, indent=4))
Conclusion
Leveraging the Pocket Option API with Python can significantly enhance your trading experience by automating various tasks and providing access to vital market data. With the examples provided in this article, you can start building your own trading bots and implement custom strategies that fit your trading style. As you delve deeper into the capabilities of the Pocket Option API, consider exploring additional features such as managing your portfolio, handling trade histories, and optimizing trading algorithms. Happy trading!